\n Definition for Python
In Python, \n is a type of escape character that will create a new line when used. There are a few other escape sequences, which are simple ways to change how certain characters work in print statements or strings. These include \t, which will tab in your text, and \", which will add a quotation in your print statement.
Here is a visual summary:
To explain in detail, one of Python’s primitive data types is called the string, or str, which is used to represent text data.
Strings are variables that can hold data besides numbers, including words. When creating string variables, their value must be inside quotation marks, like this: a_word = "between quotes"
We can create a string variable by assigning a variable text that is enclosed in either single quotes or in double quotes. (Generally, there is no difference between strings created with single quotes and with double quotes.)
doughnut_name = "Kepler"
print(doughnut_name)
Going back to the points above, escape characters can be used to help format string output.
That said, you can make a multi-line string with three quotation marks. (You won't need \n characters with this option!)
my_multiple_line_string = """This is the first line
This is the second line
This is the third line"""
To make sure we are on the same page, when we want Python to output a string to the console we use the print() function. The print() function takes a value, tries to convert it to a string if it isn’t one already, and then writes it. We can use this to create entire applications that are used exclusively from the command line.
Running this code will generate the following output in the console:
Some programming languages have different data types for single characters and for multi-character strings. Although Python doesn’t have a separate data type for individual characters, internally a string is stored as an array of characters.
We can use a for loop to print each character in a string using the print() function.
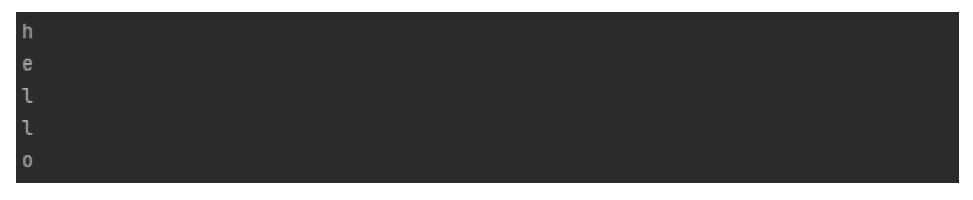
Now, in the early days of computing - before web browsers or desktop applications - there was the command line and even decades later, command line applications are still some of the most powerful tools a Python programmer can create.
The newline character, represented by \n, is a special character that is used when we need to end the current line and start a new one. The backslash in \n is called the escape character and it is always followed by a letter to create an escape sequence that is treated as a single character.
The len() function can be used to verify that \n is treated as a single character.

How to Print a New Line
Now that we know what /n means, the rest of this article will walk you through everything you need to know about printing new lines in Python to make sure your program’s output is properly formatted and readable.




How to Print Without Adding a New Line
There are scenarios where you don’t want python to automatically add a new line at the end of a print statement. Thankfully Python gives us a way to change the default print() behavior.
The print() function has an optional keyword argument named end that lets us choose how we end each line. If we don’t want a new line to be printed we can just set the end value to an empty string. This can be handy when you want to print multiple variables on the same line.
This would give us the following output:
‘\n’ Versus ‘\r\n’
If you’ve worked with newlines on Windows then you might be familiar with the \r escape sequence. This is the carriage return and it tells the computer to move the cursor to the beginning of the current line. When combined with the newline escape sequence it moves the cursor to the beginning of a new line. On Unix systems like Mac and Linux, \n performs both of these steps, so you don’t need to add a carriage return.
Writing code that runs on both Windows and Unix systems always has challenges but thankfully worrying about printing \n versus \r\n isn’t one of them. The print() function is smart enough to convert \n to \r\n when running on Windows.
Using os.linesep
The print() function may convert \n to \r\n for us, but we won’t always be using print(). Sometimes we need to write to files in binary mode, and when we do that Python won’t make any substitutions for us.
The built-in os module contains a linesep value that can be used to obtain the newline character(s) for the current system. We can use this along with the string’s replace() function to replace \n with whatever the current system’s new line character is: encode(“utf-8”) is used to convert the string into bytes so we can write it to a binary file.
If we open the file we can see it contains the following:
Wrapping Up!
The newline character, denoted by \n, is used to print a newline in Python. The print() function automatically adds a new line character at the end of its output, but this can be changed setting the end keyword argument to an empty string. Windows uses the carriage return in addition to the newline character, denoted by \r\n, but Python’s print() function converts \n to \r\n as needed.
If print()’s automatic newline conversion isn’t available, os.linesep can be used with a string’s replace() function to convert newline characters manually.
Want to learn more? Check out our Python summer camps and online learning options.